- Đăng vào
STM32 GNU MCU Eclipse - Giao tiếp USART
Giới thiệu
Tiếp tục với các tutorial lập trình trên kit STM32F4 với bộ công cụ GNU MCU Eclipse, lần này mình sẽ giới thiệu cách lập trình module USART sử dụng bộ thư viện STM32F4 HAL và thiết lập mode Debug và Release. Để biết cách cài đặt bộ công cụ GNU MCU Eclipse vui lòng xem lại bài viết này.
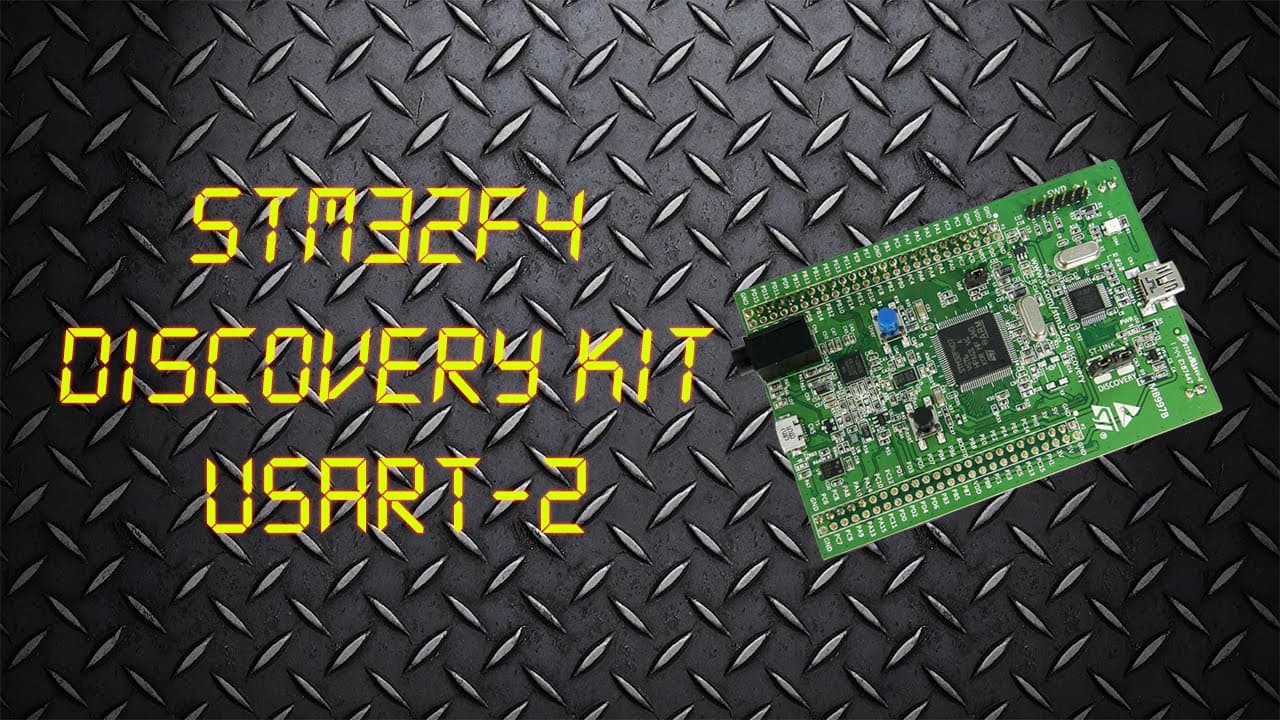
Thiết bị sử dụng:
- Kit STM32F4 Discovery đã được cài Firmware hỗ trợ J-link debug.
- Module USB UART CP2102
Code
/**
* GNU MCU Eclipse project example for USART STM32F4 Discovery
* @author nvtienanh
* @email me@nvtienanh.info
* @website https://bmshop.vn
* @ide GNU MCU Eclipse
*/
/* Include core modules */
#include #include #include "diag/Trace.h"
#include "stm32fxxx_hal.h"
#include "defines.h"
#include "stm32_disco.h"
#include "stm32_delay.h"
#include "stm32_general.h"
#include "stm32_usart.h"
// ----- main() ---------------------------------------------------------------
// Sample pragmas to cope with warnings. Please note the related line at
// the end of this function, used to pop the compiler diagnostics status.
#pragma GCC diagnostic push
#pragma GCC diagnostic ignored "-Wunused-parameter"
#pragma GCC diagnostic ignored "-Wmissing-declarations"
#pragma GCC diagnostic ignored "-Wreturn-type"
char mesg[20];
int main(int argc, char* argv[])
{
/* Init system clock for maximum system speed */
RCC_InitSystem();
/* Init HAL layer */
HAL_Init();
/* Initialize delay */
DELAY_Init();
/* Initialize leds on board */
DISCO_LedInit();
/* Init button */
DISCO_ButtonInit();
/* Init USART6, TX: PC6, 9600 baud */
USART_Init(USART6, USART_PinsPack_1, 115200);
#ifdef TRACE
trace_printf("System reset source: %d\n", (uint8_t)GENERAL_GetResetSource(1));
trace_printf("System core clock: %u Hz; PCLK1 clock: %u Hz\n",
GENERAL_GetClockSpeed(GENERAL_Clock_SYSCLK),
GENERAL_GetClockSpeed(GENERAL_Clock_PCLK1));
#endif
#ifdef NDEBUG
/* Get system reset source and clear flags after read */
/* You should see number which corresponds to "None", because we cleared flags in statement above */
sprintf(mesg,"System reset source: %d\n", (uint8_t)GENERAL_GetResetSource(1));
USART_Puts(USART6, mesg);
/* Get system core and PCLK1 (Peripheral Clock 1, APB1) clocks */
sprintf(mesg,"System core clock: %u Hz; PCLK1 clock: %u Hz\n",
GENERAL_GetClockSpeed(GENERAL_Clock_SYSCLK),
GENERAL_GetClockSpeed(GENERAL_Clock_PCLK1)
);
USART_Puts(USART6, mesg);
#endif
while (1)
{
/* If button pressed */
if (DISCO_ButtonOnPressed())
{
DISCO_LedOn(LED_ALL);
#ifdef TRACE
trace_printf("Button is pressed ...\n");
#endif
#ifdef NDEBUG
/* Send to USER */
sprintf(mesg,"Button is pressed ...\n");
USART_Puts(USART6, mesg);
#endif
/* Wait a little */
Delayms(500);
/* Perform system software reset */
GENERAL_SystemReset();
}
}
}
#pragma GCC diagnostic pop
// ----------------------------------------------------------------------------
Đoạn chương trình trên thưc hiện: khi nút nhấn được ấn, đèn led sẽ nháy và truyền dữ liệu lên máy tính theo chuẩn USART. Trong quá trình debug thì dữ liệu được hiện lên tab debug.