- Đăng vào
STM32 GNU MCU Eclipse - GPIO Led và Button
Giới thiệu
Ở bài viết trước, mình đã giới thiệu cách cài các công cụ để develop chip STM32 trên bộ công cụ GNU MCU Eclipse. Bài viết này sẽ hướng dẫn lập trình một ví dụ đơn giản về GPIO đó là nút nhấn và nháy led. Ví dụ này sử dụng: thư viện STM32 HAL mình lấy từ trang https://stm32f4-discovery.net/ và có chỉnh sửa theo mục đích cá nhân và phần cứng là kit STM32F4 Discovery. Để thực hiện thì cần Download thư viện STM32 HAL và project STM32Fxxx_DISCO.
Cấu hình project
- Bước 1: Tạo một thư mục STM32_Workspace
- Bước 2: Copy và giải nén 2 file vừa download vào thư mục trên
- Bước 3: Tạo 1 workspace tại thư mục STM32_Workspace Mở Eclipse lên vào File > Switch Workspace
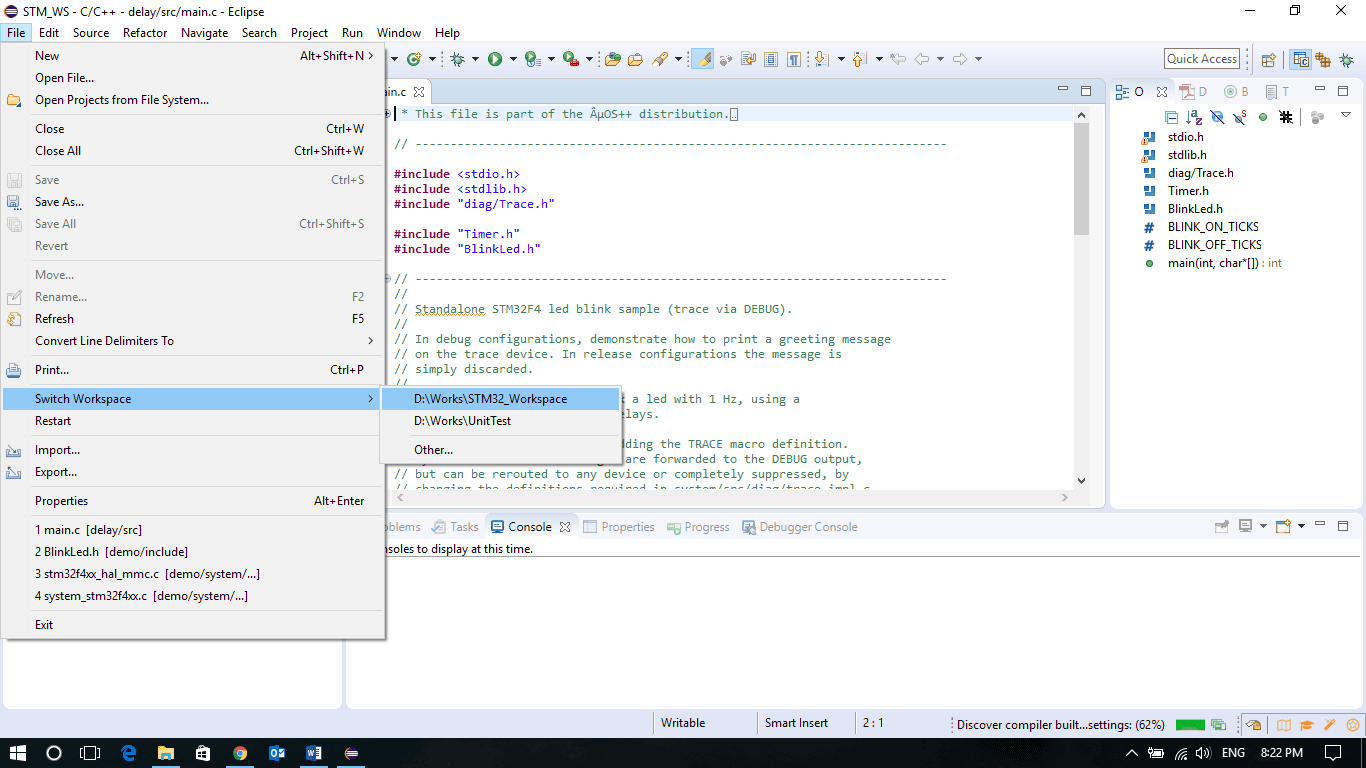
- Bước 4: Import project File > Import > Existing Project to Workspace
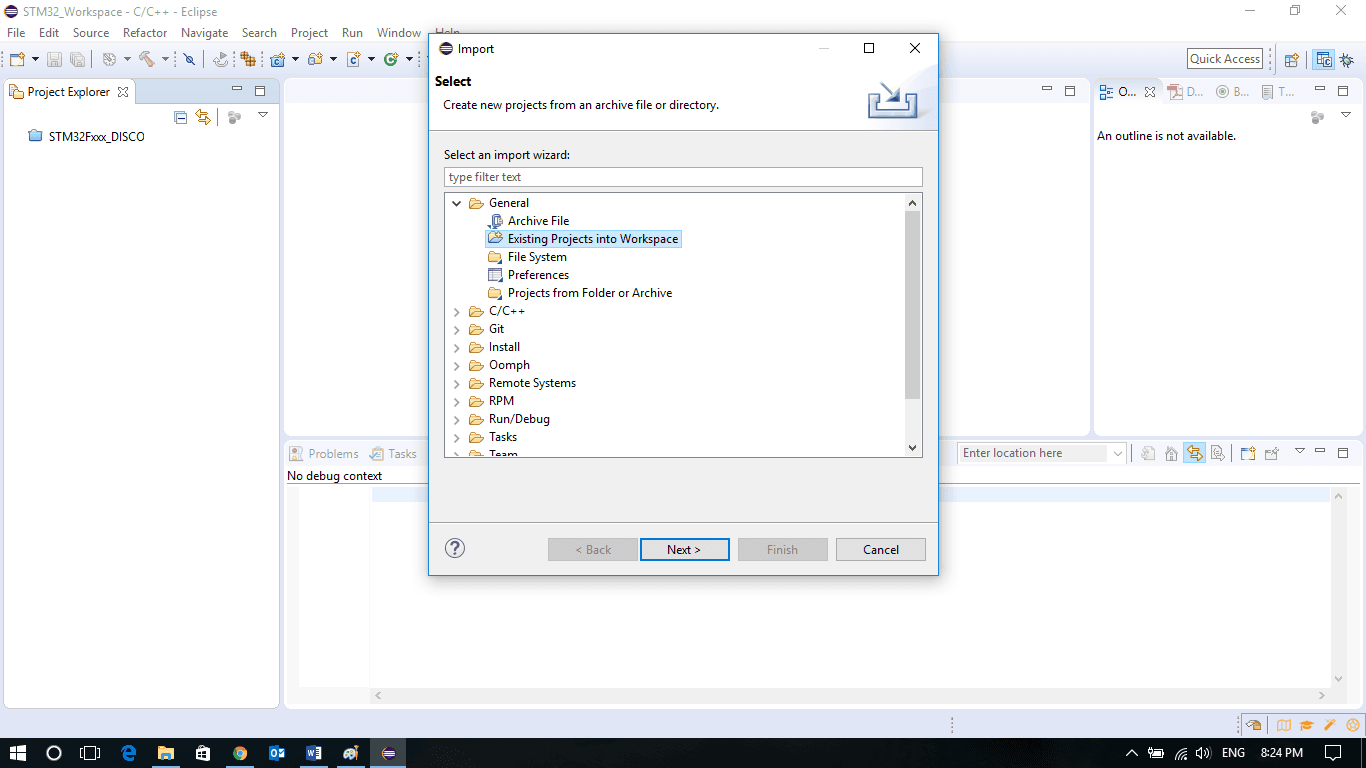
Chọn Next và click Browser… và chọn thư mục STM32Fxxx_DISCO Sau khi import xong, click chuột phải vào thư muc STM32Fxxx_DISCO ở tab Project Explorer chọn C/C++ General > Path and Symbols và click button Edit để sửa đường dẫn của thư viện STM32 HAL
Lưu ý: sửa cho cả 3 languages: Assembly, GNU C và GNU C++
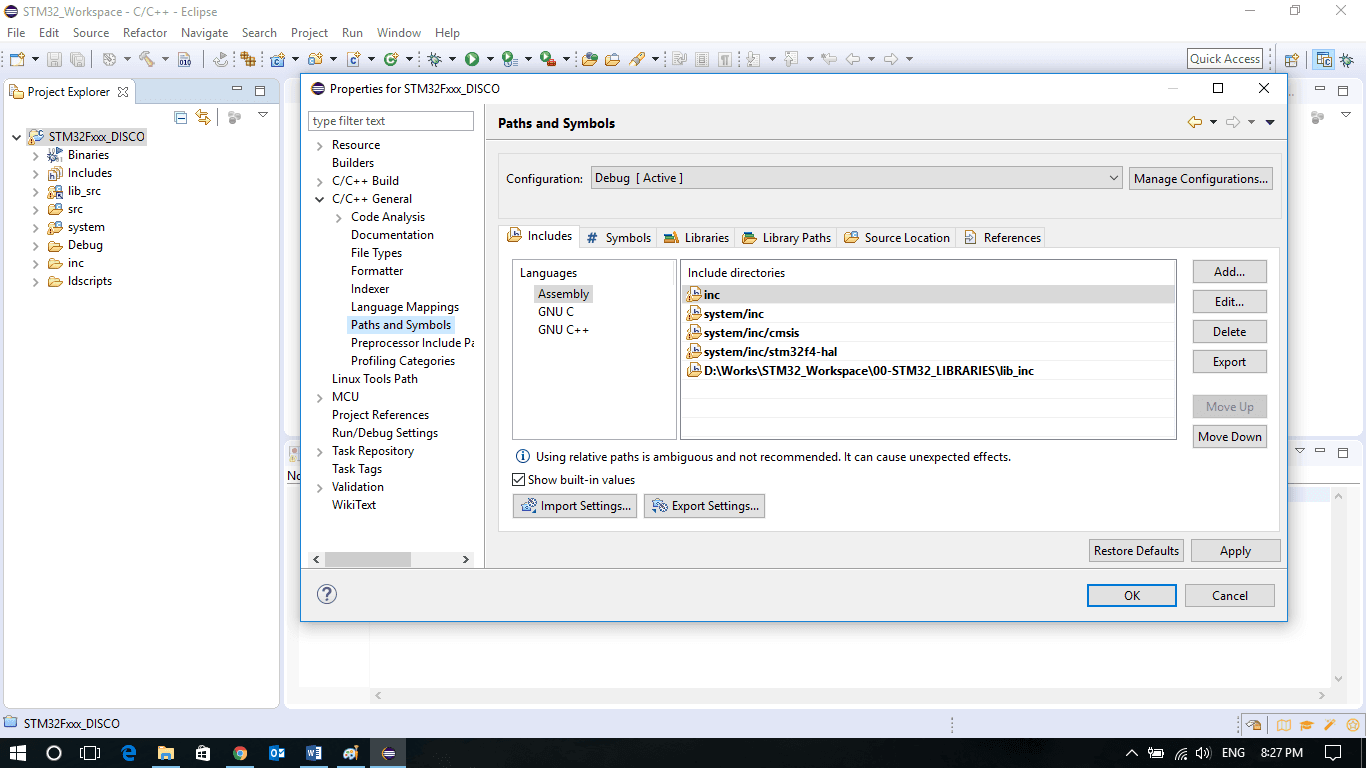
- Bước 5: Build project Build (Debug) project thành công sẽ có được kết quả như hình
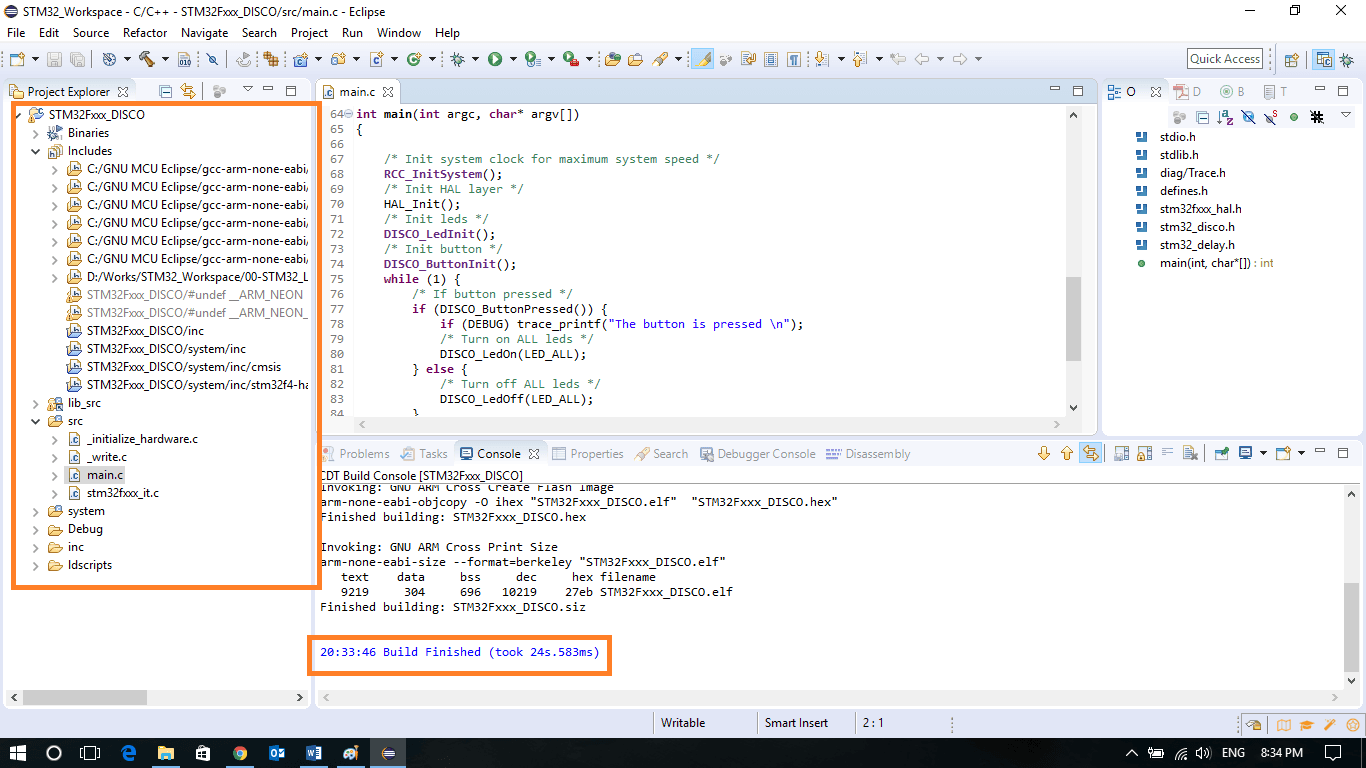
- Bước 6: Cấu hình Debug Click chuột phải vào vào thư muc STM32Fxxx_DISCO ở tab Project Explorer chọn Debug as > Debug Configuration… rồi double click vào GDB SEGGER J-Link Debugging
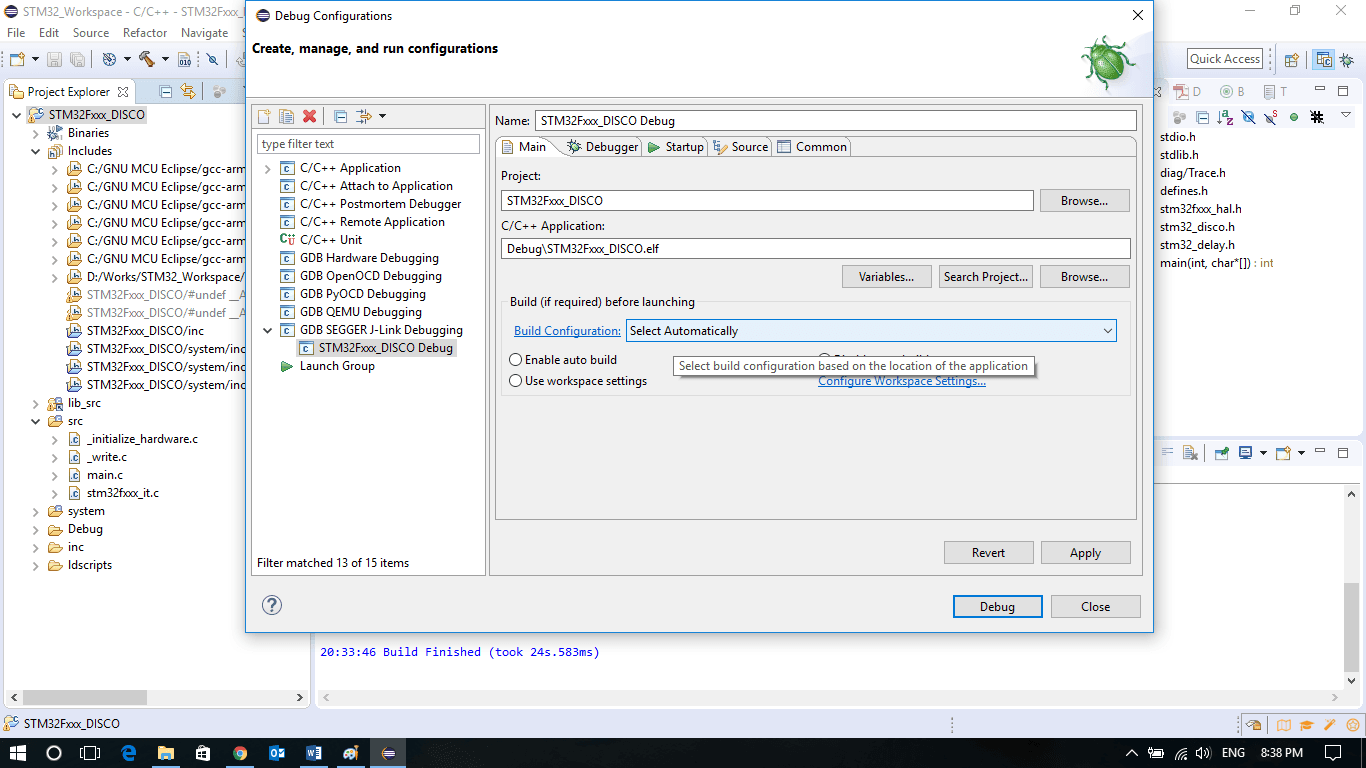
Sau đo click Apply rồi click Debug, giao diện Debug sẽ hiện ra
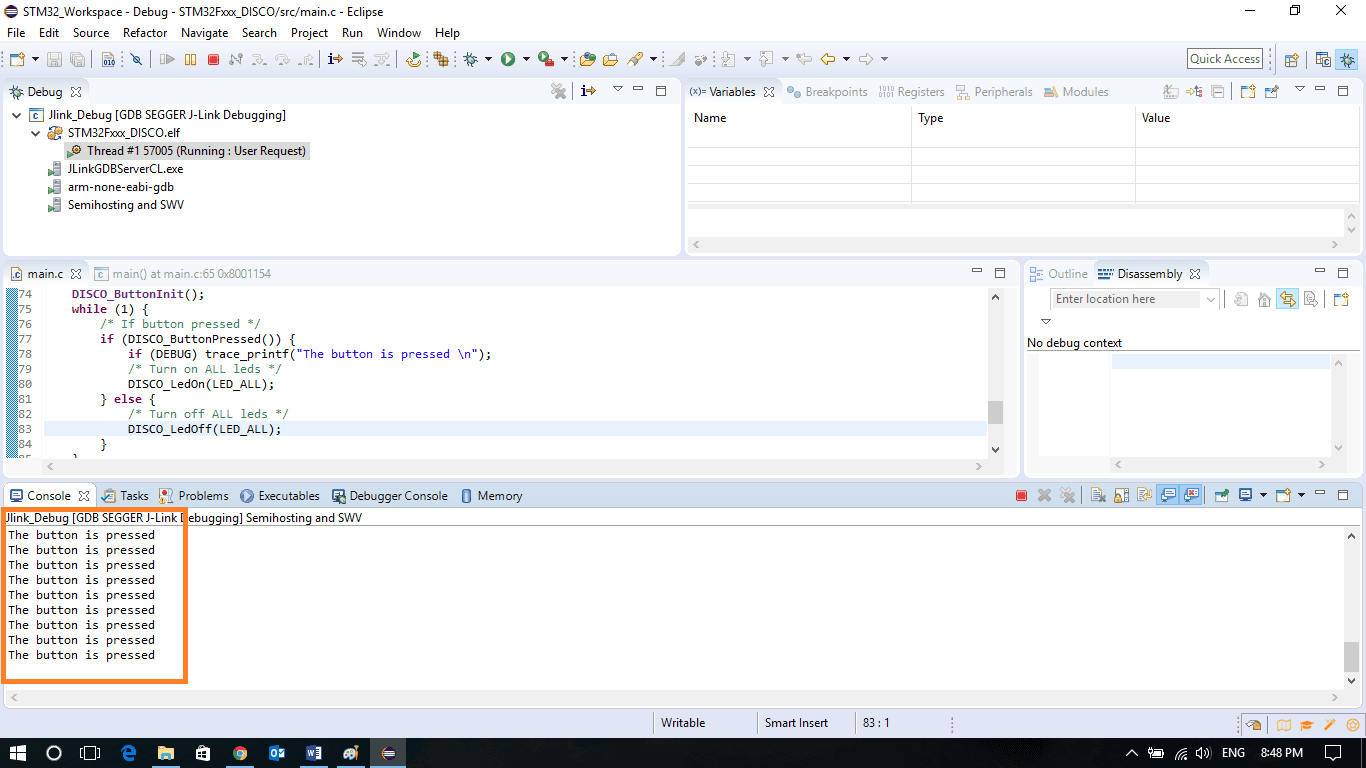
Ấn F8 để bắt đầu debug Khi bạn ấn vào button trên kit thì ở tab console bên dưới sẽ hiện Khi muốn ngừng debug thì bạn chỉ cần click button stop. Đây chỉ là ví dụ đơn giản, dựa trên đó các bạn có thể phát triển them các ứng dụng khác và dung chức năng Debug kể kiểm tra kết quả.
Khi bạn nhấn nút trên board thì ở Console hiện dòng chữ: The button is pressed là bởi vì trong file main.c line 78, khi nút được ấn thì sẽ print message ra console.
/*
* This file is part of the µOS++ distribution.
* (https://github.com/micro-os-plus)
* Copyright (c) 2014 Liviu Ionescu.
*
* Permission is hereby granted, free of charge, to any person
* obtaining a copy of this software and associated documentation
* files (the "Software"), to deal in the Software without
* restriction, including without limitation the rights to use,
* copy, modify, merge, publish, distribute, sublicense, and/or
* sell copies of the Software, and to permit persons to whom
* the Software is furnished to do so, subject to the following
* conditions:
*
* The above copyright notice and this permission notice shall be
* included in all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
* EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
* OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
* NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
* HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
* WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
* FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
* OTHER DEALINGS IN THE SOFTWARE.
*/
// ----------------------------------------------------------------------------
#include <stdio.h>
#include <stdlib.h>
#include "diag/Trace.h"
#include "defines.h"
#include "stm32fxxx_hal.h"
#include "stm32_disco.h"
#include "stm32_delay.h"
// ----------------------------------------------------------------------------
//
// Standalone STM32F4 led blink sample (trace via DEBUG).
//
// In debug configurations, demonstrate how to print a greeting message
// on the trace device. In release configurations the message is
// simply discarded.
//
// Then demonstrates how to blink a led with 1 Hz, using a
// continuous loop and SysTick delays.
//
// Trace support is enabled by adding the TRACE macro definition.
// By default the trace messages are forwarded to the DEBUG output,
// but can be rerouted to any device or completely suppressed, by
// changing the definitions required in system/src/diag/trace_impl.c
// (currently OS_USE_TRACE_ITM, OS_USE_TRACE_SEMIHOSTING_DEBUG/_STDOUT).
//
// ----- main() ---------------------------------------------------------------
// Sample pragmas to cope with warnings. Please note the related line at
// the end of this function, used to pop the compiler diagnostics status.
#pragma GCC diagnostic push
#pragma GCC diagnostic ignored "-Wunused-parameter"
#pragma GCC diagnostic ignored "-Wmissing-declarations"
#pragma GCC diagnostic ignored "-Wreturn-type"
int main(int argc, char* argv[])
{
/* Init system clock for maximum system speed */
RCC_InitSystem();
/* Init HAL layer */
HAL_Init();
/* Init leds */
DISCO_LedInit();
/* Init button */
DISCO_ButtonInit();
while (1) {
/* If button pressed */
if (DISCO_ButtonPressed()) {
if (DEBUG) trace_printf("The button is pressed \n");
/* Turn on ALL leds */
DISCO_LedOn(LED_ALL);
} else {
/* Turn off ALL leds */
DISCO_LedOff(LED_ALL);
}
}
}
#pragma GCC diagnostic pop
// ----------------------------------------------------------------------------