- Đăng vào
STM32 GNU MCU Eclipse: Timer callback function
- Tác giả
- Tên
- Anh Nguyễn
- @nvtienanh
Bài viết này giới thiệu lập trình sử dụng chức năng đếm timer, trong ví dụ demo dưới đây cứ sau 100ms, đèn led xanh sẽ nháy sáng 1 lần và cứ sau 1s đèn đỏ sẽ nháy 1 lần. Dĩ nhiên là thay vì nháy led chúng ta có thể làm việc khác như tính toán xử lý số liệu đọc cảm biến thay vì nháy led.
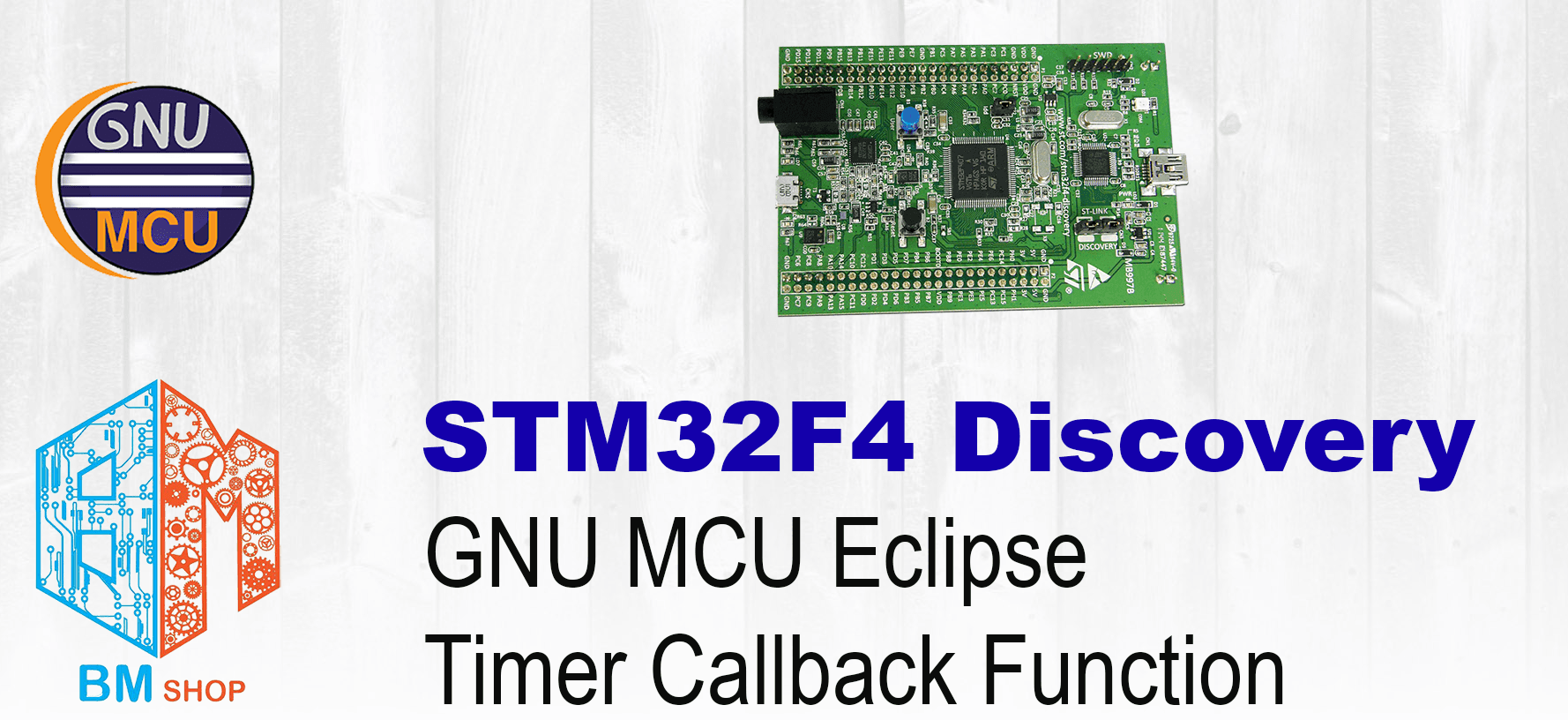
Các bạn có thể download Project mẫu GNU MCU Eclipse ở cuối bài viết. Dưới đây là code file main.c để mọi người có thể hình dung được cấu trúc của chướng trình. Cuối bài viết là Project dùng timer, để download toàn bộ thư viện và các file header các bạn vui lòng xem bài viết này.
/**
* GNU MCU Eclipse project example for HD44780
*
* Before you start, select your target, on the right of the "Load" button
*
* @author nvtienanh
* @email me@nvtienanh.info
* @website https://bmshop.vn
* @ide GNU MCU Eclipse
* @stdperiph STM32F4xx/STM32F7xx HAL drivers required
*/
/* Include core modules */
#include <stdio.h>
#include <stdlib.h>
#include "diag/Trace.h"
#include "stm32fxxx_hal.h"
#include "defines.h"
#include "stm32_disco.h"
#include "stm32_delay.h"
// ----- main() ---------------------------------------------------------------
// Sample pragmas to cope with warnings. Please note the related line at
// the end of this function, used to pop the compiler diagnostics status.
#pragma GCC diagnostic push
#pragma GCC diagnostic ignored "-Wunused-parameter"
#pragma GCC diagnostic ignored "-Wmissing-declarations"
#pragma GCC diagnostic ignored "-Wreturn-type"
/* Create pointers for 2 timers */
DELAY_Timer_t* SWTIM1;
DELAY_Timer_t* SWTIM2;
/* References for function callbacks */
void SWTIM1_Callback(DELAY_Timer_t* SWTIM, void* UserParameters);
void SWTIM2_Callback(DELAY_Timer_t* SWTIM, void* UserParameters);
int main(int argc, char* argv[])
{
/* Init system clock for maximum system speed */
RCC_InitSystem();
/* Init HAL layer */
HAL_Init();
/* Init leds */
DISCO_LedInit();
/* Init button */
DISCO_ButtonInit();
/* Init delay */
DELAY_Init();
/* Create software timers */
/* 100ms with autoreload enabled, and start after it is created */
SWTIM1 = DELAY_TimerCreate(100, 1, 1, SWTIM1_Callback, NULL);
/* 1s with autoreload enabled, and start after it is created */
SWTIM2 = DELAY_TimerCreate(1000, 1, 1, SWTIM2_Callback, NULL);
while (1)
{
#ifdef TRACE
// For Debug
#endif
#ifdef NDEBUG
// For Release
#endif
}
}
/* Callback function for SWTIM1 */
void SWTIM1_Callback(DELAY_Timer_t* SWTIM, void* UserParameters) {
/* Toggle green led */
DISCO_LedToggle(LED_GREEN);
}
/* Callback function for SWTIM2 */
void SWTIM2_Callback(DELAY_Timer_t* SWTIM, void* UserParameters) {
/* Toggle red led */
DISCO_LedToggle(LED_RED);
}