- Đăng vào
Truyền tín hiệu lên máy tính theo chuẩn RS232
Ở bài trước mình đã giới thiệu cách đọc tín hiệu từ cảm biến MPU6050 theo chuẩn I2C dung vi điều khiển PIC, bây giờ chúng ta sẽ truyền tín hiệu đó lên máy tính theo chuẩn RS232 vẽ đồ thị góc quay theo thời gian. Giao diện được lập trình bằng C# Các thông số của giao diện
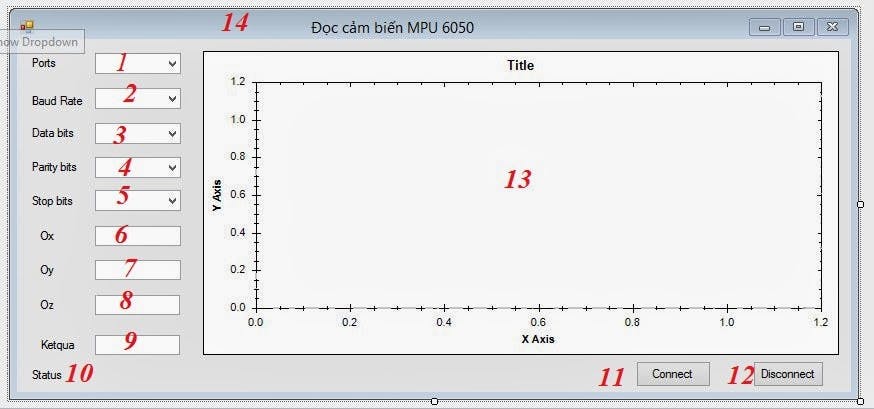
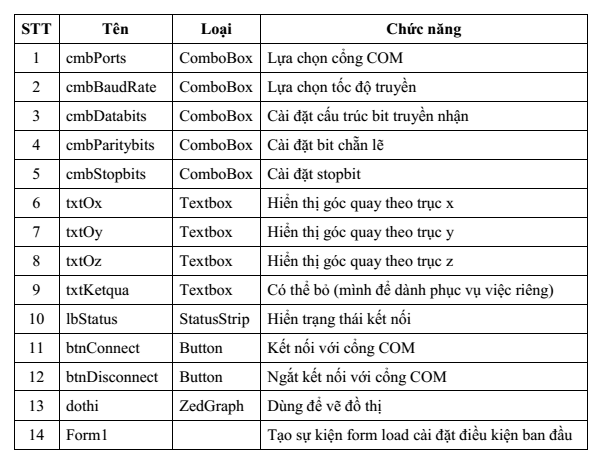
Xử lý sự kiện
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
// Khai bao thu vien Seiral Ports
using System.Windows;
using System.IO;
using System.IO.Ports;
using System.Xml;
using ZedGraph;
namespace Giao_tiep_RS232
{
public partial class Form1 : Form
{
// Khoi tao doi tuong Serial Ports P
SerialPort P = new SerialPort();
Boolean PortOpen = false;
string InputData = string.Empty; // khai bao buffer dem kieu string
string temp = string.Empty;
string lines = "rn Gryo_Xt Gryo_Yt Gryo_Zt Acce_Xt Acce_Yt Acce_Zt rn";
StreamWriter file = new StreamWriter("E:\_MPU6050_.txt");
delegate void SetTextCallBack(String Text); // Khai bao thu tuc delegate
int tickStart = 0;
float Ox;
public Form1()
{
InitializeComponent();
string[] ports = SerialPort.GetPortNames();// Liet ke cac ports tim thay
cmbPorts.Items.AddRange(ports);// Them cac cong tim thay vao Combobox
P.ReadTimeout = 1000;
P.DataReceived += new SerialDataReceivedEventHandler(DataReceive);
// Khoi tao Baudrate
string[] Baudrate = {"1200","2400","4800","9600","19200","38400","57600","115200" };
cmbBaudRate.Items.AddRange(Baudrate);
//Khoi tao data bits
string[] Databits = {"6","7","8"};
cmbDatabits.Items.AddRange(Databits);
// Khoi tao Parity bit
string[] Paritybits = {"None","Odd","Even"};
cmbParitybits.Items.AddRange(Paritybits);
// Khoi tao Stop bit
string[] Stopbits = {"1","1.5","2"};
cmbStopbits.Items.AddRange(Stopbits);
}
// Xu ly su kien khi lua chon cac thong so cua he thong
private void cmbPorts_SelectedIndexChanged(object sender, EventArgs e)
{
if (P.IsOpen) P.Close();
P.PortName = cmbPorts.SelectedItem.ToString();
}
private void cmbBaudRate_SelectedIndexChanged(object sender, EventArgs e)
{
if (P.IsOpen) P.Close();
P.BaudRate = Convert.ToInt32(cmbBaudRate.Text); // Chuyen kieu String Sang kieu so nguyen
}
private void cmbDatabits_SelectedIndexChanged(object sender, EventArgs e)
{
if (P.IsOpen) P.Close();
P.DataBits = Convert.ToInt32(cmbDatabits.Text);
}
private void cmbParitybits_SelectedIndexChanged(object sender, EventArgs e)
{
if (P.IsOpen) P.Close();
switch (cmbParitybits.SelectedItem.ToString())
{
case "Odd":
P.Parity = Parity.Odd;
break;
case "None":
P.Parity = Parity.None;
break;
case "Even":
P.Parity = Parity.Even;
break;
}
}
private void cmbStopbits_SelectedIndexChanged(object sender, EventArgs e)
{
if (P.IsOpen) P.Close();
switch (cmbStopbits.SelectedItem.ToString())
{
case "1":
P.StopBits = StopBits.One;
break;
case "1.5":
P.StopBits = StopBits.OnePointFive;
break;
case "2":
P.StopBits = StopBits.Two;
break;
}
}
// Xu ly truyen nhan du lieu qua cong COM
private void DataReceive(object sender, SerialDataReceivedEventArgs e)
{
if (PortOpen)
{
// Tach chuoi gia tri
InputData = P.ReadLine();
for (int i = 0; i < InputData.Length; i++)
{
// Nen sua lai thanh cau truc switch case: // Tien Anh 24/2/2014
if (InputData[i] == 'a')
{
SetTextOx(temp);
lines += (temp + "t");
//Ox = Convert.ToDouble(temp);
Ox = float.Parse(temp);
temp = string.Empty;
continue;
}
else
if (InputData[i] == 'y')
{
SetTextOy(temp);
lines += (temp + "t");
temp = string.Empty;
continue;
}
else
if (InputData[i] == 'z')
{
SetTextOz(temp);
lines += (temp + "trn");
file.WriteLine(lines);
lines = string.Empty;
temp = string.Empty;
continue;
}
temp += InputData[i];
}
}
}
// Ham SetText
private void SetText(string text)
{
if (this.txtKetqua.InvokeRequired)
{
SetTextCallBack d = new SetTextCallBack(SetText);// Khoi tao 1 delegate goi den SetText
this.BeginInvoke(d, new object[] { text });
}
else
{//this.txtKetqua.Text += text;
this.txtKetqua.Clear();
this.txtKetqua.Text = text; // Tu dong Refresh man hinh
}
}
// Ham SetTextADC
private void SetTextADC(string text)
{
if (this.txtKetqua.InvokeRequired)
{
SetTextCallBack d = new SetTextCallBack(SetTextADC);// Khoi tao 1 delegate goi den SetText
this.BeginInvoke(d, new object[] { text });
}
else
{//this.txtKetqua.Text += text;
this.txtKetqua.Clear();
this.txtKetqua.Text += text; // Tu dong Refresh man hinh
}
}
// Ham SetTextOx
private void SetTextOx(string text)
{
if (this.txtKetqua.InvokeRequired)
{
SetTextCallBack d = new SetTextCallBack(SetTextOx);// Khoi tao 1 delegate goi den SetText
this.BeginInvoke(d, new object[] { text });
}
else
{//this.txtKetqua.Text += text;
this.txtOx.Clear();
this.txtOx.Text += text; // Tu dong Refresh man hinh
}
}
// Ham SetTextOy
private void SetTextOy(string text)
{
if (this.txtKetqua.InvokeRequired)
{
SetTextCallBack d = new SetTextCallBack(SetTextOy);// Khoi tao 1 delegate goi den SetText
this.BeginInvoke(d, new object[] { text });
}
else
{//this.txtKetqua.Text += text;
this.txtOy.Clear();
this.txtOy.Text += text; // Tu dong Refresh man hinh
}
}
// Ham SetTextOz
private void SetTextOz(string text)
{
if (this.txtKetqua.InvokeRequired)
{
SetTextCallBack d = new SetTextCallBack(SetTextOz);// Khoi tao 1 delegate goi den SetText
this.BeginInvoke(d, new object[] { text });
}
else
{//this.txtKetqua.Text += text;
this.txtOz.Clear();
this.txtOz.Text += text; // Tu dong Refresh man hinh
}
}
private void textBox1_TextChanged(object sender, EventArgs e)
{
}
// Khoi tao gia tri ban dau khi chay chuong trinh
private void Form1_Load(object sender, EventArgs e)
{
cmbPorts.SelectedIndex = 2;
cmbBaudRate.SelectedIndex = 4;
cmbDatabits.SelectedIndex = 2;
cmbParitybits.SelectedIndex = 0;
cmbStopbits.SelectedIndex = 0;
lbStatus.Text = "Port is closed!";
txtKetqua.Text = "";
btnDisconnect.Enabled = false;
// Khoi tao ve do thi
GraphPane myPane = dothi.GraphPane;
myPane.Title.Text = "Đọc tín hiệu từ cảm biến MPU 6050";
myPane.XAxis.Title.Text = "Time , seconds";
myPane.YAxis.Title.Text = "Góc quay, deg";
// Save 1200 points. At 50 ms sample rate, this is one minute
// The RollingPointPairList is an efficient storage class that always
// keeps a rolling set of point data without needing to shift any data values
RollingPointPairList list = new RollingPointPairList(60000);
// Initially, a curve is added with no data points (list is empty)
// Color is blue, and there will be no symbols
LineItem curve = myPane.AddCurve("Roll Angle", list, Color.Blue, SymbolType.None);
// Sample at 1 ms intervals
timer1.Interval = 2;
timer1.Enabled = true;
// Just manually control the X axis range so it scrolls continuously
// instead of discrete step-sized jumps
myPane.XAxis.Scale.Min = 0;
myPane.XAxis.Scale.Max = 20;
myPane.XAxis.Scale.MinorStep = 1;
myPane.XAxis.Scale.MajorStep = 5;
myPane.YAxis.Scale.Min =-90;
myPane.YAxis.Scale.Max = 90;
// Scale the axes
dothi.AxisChange();
// Save the beginning time for reference
tickStart = Environment.TickCount;
//dudPWM.Text = "0";
}
// Xu ly su kien Click nut Connect
private void btnConnect_Click(object sender, EventArgs e)
{
try
{
P.Open();
PortOpen = true;
btnDisconnect.Enabled = true;
btnConnect.Enabled = false;
lbStatus.Text = "Port is connecting with " + cmbPorts.SelectedItem.ToString();
timer1.Start();
}
catch (Exception ex )
{
MessageBox.Show("Not connect","Try",MessageBoxButtons.OK,MessageBoxIcon.Error);
}
file.WriteLine(lines);
lines = string.Empty;
}
// Xu ly su kien Click nut Disconnect
private void btnDisconnect_Click(object sender, EventArgs e)
{
file.Close();
P.Close();
PortOpen = false;
btnConnect.Enabled = true;
btnDisconnect.Enabled = false;
lbStatus.Text = "Port is closed!";
}
// Xu ly su kien timer dem xong
private void timer1_Tick(object sender, EventArgs e)
{
// Make sure that the curvelist has at least one curve
if (dothi.GraphPane.CurveList.Count <= 0) return; // Get the first CurveItem in the graph LineItem curve = dothi.GraphPane.CurveList[0] as LineItem; if (curve == null) return; // Get the PointPairList IPointListEdit list = curve.Points as IPointListEdit; // If this is null, it means the reference at curve.Points does not // support IPointListEdit, so we won't be able to modify it if (list == null) return; // Time is measured in seconds double time = (Environment.TickCount - tickStart) / 1000.0; // 3 seconds per cycle if (txtOx.Text != null) { //double temp; // temp = double.Parse(txtOx.Text); list.Add(time, Ox); } // Keep the X scale at a rolling 30 second interval, with one // major step between the max X value and the end of the axis Scale xScale = dothi.GraphPane.XAxis.Scale; if (time > xScale.Max - xScale.MajorStep)
{
xScale.Max = time + xScale.MajorStep;
xScale.Min = xScale.Max - 20.0;
}
// Make sure the Y axis is rescaled to accommodate actual data
dothi.AxisChange();
// Force a redraw
dothi.Invalidate();
}
}// End public partial class Form1 : Form
}