- Đăng vào
Đọc và lưu giá trị cảm biệt nhiệt độ, độ ẩm vào thẻ nhớ
Giới thiệu
Trong bài viết này sẽ hướng dẫn đọc cảm biến nhiệt độ, độ ẩm tương đối và lưu trữ dữ liệu vào thẻ nhớ bằng Arduino. Cảm biến được sử dụng là DHT22 dùng để đo cả nhiệt độ và độ ẩm tương đối, board Arduino được sử dụng là Uno, ngoài ra dữ liệu cảm biến được lưu vào thẻ nhớ bằng modun SD Card. Dữ liệu từ thẻ nhớ được import vào Excel để vẽ đồ thị.
Danh sách thiết bị
- Arduino Uno
- Modun SD Card
- Cảm biến DHT22 đo nhiệt độ và độ ẩm
- Thẻ nhớ ( dung lượng > 8GB)
- Board cắm dây
- Dây cắm
Sơ đồ đấu dây
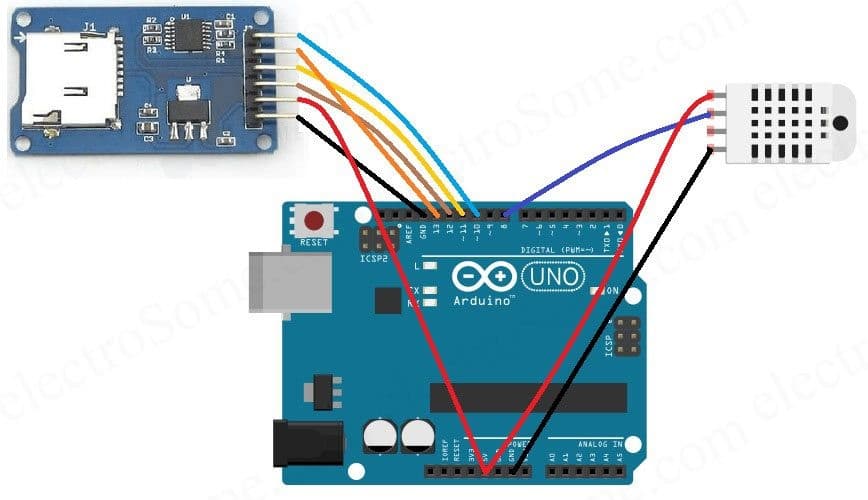
Nối SD Card với Arduino
Đầu tiên, chúng ta kết nối modun SD Card với Arduino, modun SD Card được đọc và ghi dữ liệu bằng giao tiếp SPI vì vậy chúng ta cần kết nối các chân:
- Chân GND SD Card với chân GND của Arduino Uno
- Chân VCC của modun SD Card với chân ouput 5V của Arduino Uno
- Chân MISO của modun SD Card với chân 12 của Arduino Uno
- Chân MOSI của modun SD Card với chân 11 của Arduino Uno
- Chân SCK của modun SD Card với chân 13 của Arduino Uno
- Chân CS của modun SD Card với chân 10 của Arduino Uno
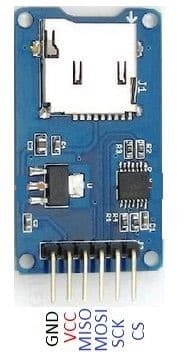
Cảm biến DHT22 Arduino
Tiếp theo chúng ta kết nối cảm biến DHT22 với Arduino Uno.
- Chân VCC của DHT22 với 5V output của Arduino Uno
- Chân GND của DHT22 với chân GND của Arduino Uno
- Chân Data của DHT22 với chân 8 của Arduino Uno
Hình ảnh thực tế sau khi kết nối
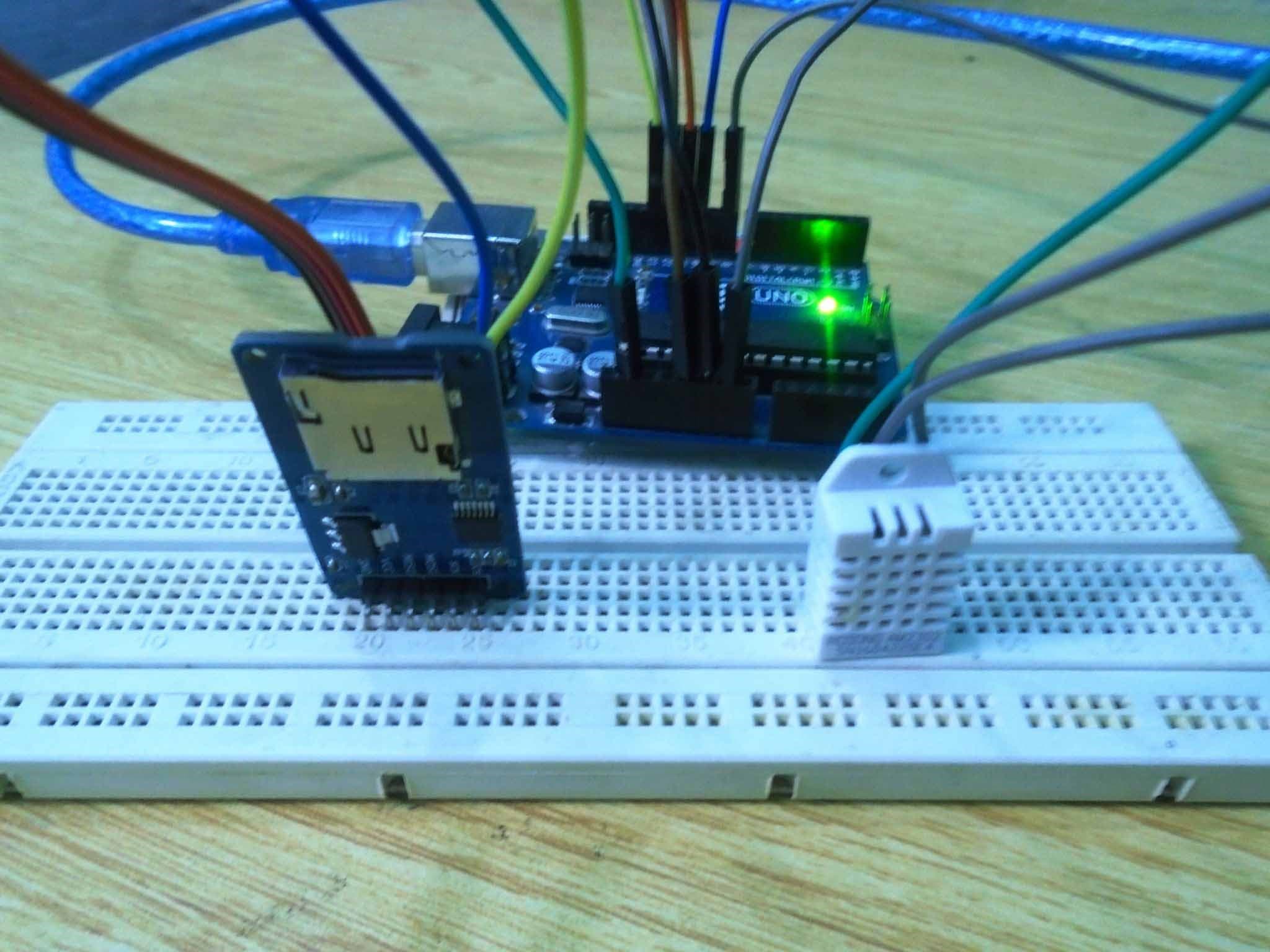
Code Arduino
Thêm thư viện DHT22 vào Arduino
- Download thư viện DHT tại : DHT Sensor Library.
- Mở Arduino IDE.
- Vào menu Sketch >> Include Library >> Add .zip Library
- Chọn file .zip và bạn vừa download.
#include <SD.h>
#include <SPI.h>
#include "DHT.h"
#define DHTPIN 8
#define DHTTYPE DHT22
long seconds=00;
long minutes=00;
long hours=00;
int CS_pin = 10;
DHT dht(DHTPIN, DHTTYPE);
File sd_file;
void setup() {
Serial.begin(9600);
pinMode(CS_pin, OUTPUT);
dht.begin();
// SD Card Initialization
if (SD.begin()) {
Serial.println("SD card is initialized. Ready to go");
}
else {
Serial.println("Failed");
return;
}
sd_file = SD.open("data.txt", FILE_WRITE);
if (sd_file) {
Serial.print("Time");
Serial.print(",");
Serial.print("Humidity");
Serial.print(",");
Serial.print("Temperature_C");
Serial.print(",");
Serial.print("Temperature_F");
Serial.print(",");
Serial.println("Heat_index");
sd_file.print("Time");
sd_file.print(",");
sd_file.print("Humidity");
sd_file.print(",");
sd_file.print("Temperature_C");
sd_file.print(",");
sd_file.print("Temperature_F");
sd_file.print(",");
sd_file.println("Heat_index");
}
sd_file.close(); //closing the file
}
void loop() {
sd_file = SD.open("data.txt", FILE_WRITE);
if (sd_file) {
senddata();
}
// if the file didn't open, print an error:
else {
Serial.println("error opening file");
}
delay(1000);
}
void senddata() {
for(long seconds = 00; seconds < 60; seconds=seconds+2) {
float temp = dht.readTemperature(); //Reading the temperature as Celsius and storing in temp
float hum = dht.readHumidity(); //Reading the humidity and storing in hum
float fah = dht.readTemperature(true);
float heat_index = dht.computeHeatIndex(fah, hum);
sd_file.print(hours);
sd_file.print(":");
sd_file.print(minutes);
sd_file.print(":");
sd_file.print(seconds);
sd_file.print(", ");
sd_file.print(hum);
sd_file.print(", ");
sd_file.print(temp);
sd_file.print(", ");
sd_file.print(fah);
sd_file.print(", ");
sd_file.println(heat_index);
Serial.print(hours);
Serial.print(":");
Serial.print(minutes);
Serial.print(":");
Serial.print(seconds);
Serial.print(", ");
Serial.print(hum);
Serial.print(", ");
Serial.print(temp);
Serial.print(", ");
Serial.print(fah);
Serial.print(", ");
Serial.println(heat_index);
if(seconds>=58) {
minutes= minutes + 1;
}
if (minutes>59) {
hours = hours + 1;
minutes = 0;
}
sd_file.flush(); //saving the file
delay(2000);
}
sd_file.close(); //closing the file
}
Giải thích code
Khai báo thư viện và các chân kết nối
#include <SD.h> // Including SD Card Library
#include <SPI.h> // Including SPI Library
#include "DHT.h" // Including DHT Sensor Library
#define DHTPIN 8 // Declaring the pin to which DHT data pin is connected
#define DHTTYPE DHT22 // Selecting the type of DHT sensor used in this project
long seconds=00; // For storing second
long minutes=00; // For storing minute
long hours=00; // For storing hour
int CS_pin = 10; // Chip select pin of SD card module
DHT dht(DHTPIN, DHTTYPE); // Declaring DHT object
File sd_file; // Declaring File object
Setup function Đoạn code trong function setup chúng ta thực hiện các công việc
- Khởi tạo phần giao tiếp serial với baudrate là 9600, lưu ý rằng giao tiếp serial này dung để hiển thị dữ liệu lên Arduino Serial Monitor.
- dht.begin() là function trong thư viện DHT dùng để khởi tạo ban đầu modun cảm biến DHT22
- sd.begin() là function trong thư viện SD Card dùng để khởi tạo ban đầu modun SD Card
- sd_file = SD.open("data.txt", FILE_WRITE); dùng để tạo fil data.txt trên thẻ nhớ nhằm lưu dữ liệu của cảm biến
void setup() {
Serial.begin(9600);
pinMode(CS_pin, OUTPUT);
dht.begin(); // Initialize the Arduino for reading data from DHT sensor
// SD Card Initialization
if (SD.begin()) {
Serial.println("SD card is initialized. Ready to go");
}
else {
Serial.println("Failed");
return;
}
sd_file = SD.open("data.txt", FILE_WRITE);
if (sd_file) {
Serial.print("Time");
Serial.print(",");
Serial.print("Humidity");
Serial.print(",");
Serial.print("Temperature_C");
Serial.print(",");
Serial.print("Temperature_F");
Serial.print(",");
Serial.println("Heat_index");
sd_file.print("Time");
sd_file.print(",");
sd_file.print("Humidity");
sd_file.print(",");
sd_file.print("Temperature_C");
sd_file.print(",");
sd_file.print("Temperature_F");
sd_file.print(",");
sd_file.println("Heat_index");
}
sd_file.close(); //closing the file
}
Vòng lặp Dữ liệu được đọc 1 lần/s
void loop() {
sd_file = SD.open("data.txt", FILE_WRITE);
if (sd_file) {
senddata();
}
// if the file didn't open, print an error:
else {
Serial.println("error opening file");
}
delay(1000);
}
Trong chương trình chính có sử dụng function senddata() Chương trình này sẽ lưu trữ dữ liệu của cảm biến kèm ngày, giờ cụ thể function senddata()
void senddata() {
for(long seconds = 00; seconds < 60; seconds=seconds+2) {
float temp = dht.readTemperature(); //Reading the temperature as Celsius and storing in temp
float hum = dht.readHumidity(); //Reading the humidity and storing in hum
float fah = dht.readTemperature(true);
float heat_index = dht.computeHeatIndex(fah, hum);
sd_file.print(hours);
sd_file.print(":");
sd_file.print(minutes);
sd_file.print(":");
sd_file.print(seconds);
sd_file.print(", ");
sd_file.print(hum);
sd_file.print(", ");
sd_file.print(temp);
sd_file.print(", ");
sd_file.print(fah);
sd_file.print(", ");
sd_file.println(heat_index);
Serial.print(hours);
Serial.print(":");
Serial.print(minutes);
Serial.print(":");
Serial.print(seconds);
Serial.print(", ");
Serial.print(hum);
Serial.print(", ");
Serial.print(temp);
Serial.print(", ");
Serial.print(fah);
Serial.print(", ");
Serial.println(heat_index);
if(seconds>=58) {
minutes= minutes + 1;
}
if (minutes>59) {
hours = hours + 1;
minutes = 0;
}
sd_file.flush(); //saving the file
delay(2000);
}
sd_file.close(); //closing the file
}
Importing dữ liệu vào Excel
Để nhập dữ liệu cảm biến vào Excel và vẽ đồ thị chúng ta lấy thẻ nhớ khói modun và cắm vào đầu đọc thẻ nhớ vào excel và open file data.txt trong thẻ nhớ
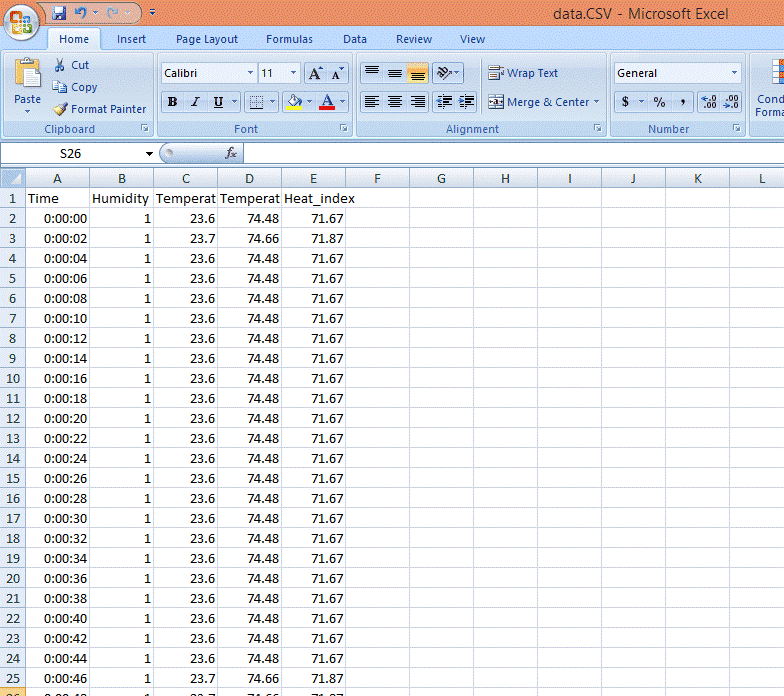
Để vẽ đồ thị trong excel chúng ta thực hiện
- Vào tab Insert
- Chọn Line option
- Chọn 2D line
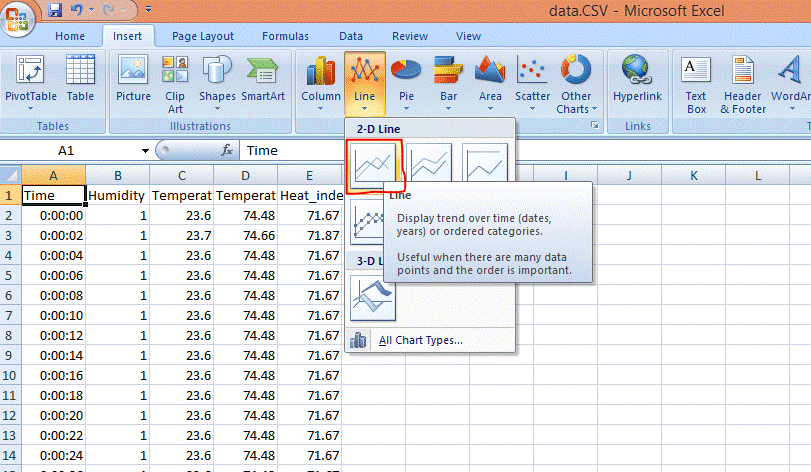
Kết quả demo
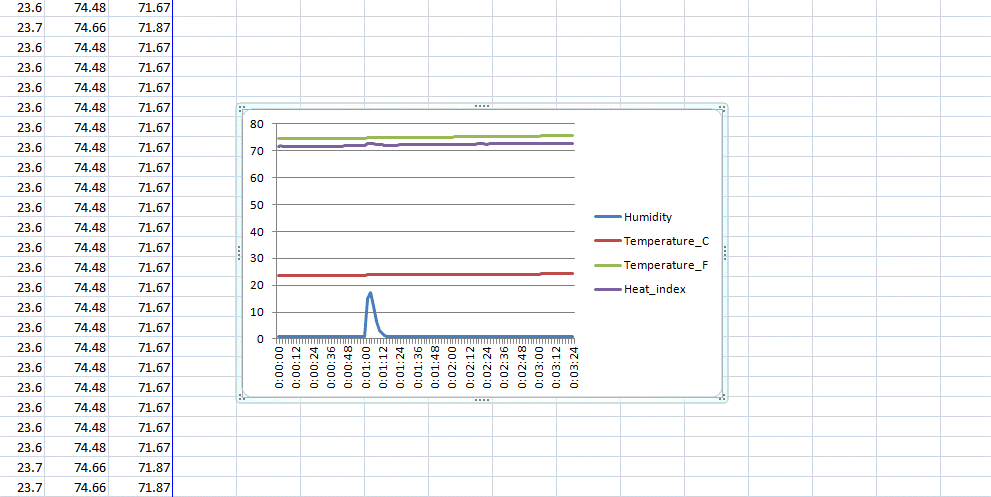