- Đăng vào
STM32 GNU MCU Eclipse: LCD HD44780
Giới thiệu
Bài viết này sẽ lập trình LCD HD44780 trên kit STM32F4 Discovery với bộ công cụ GNU MCU Eclipse. Một số tính năng của thư viện HD44780 sử dụng trong ví dụ này:
- Giao tiếp với LCD ở chế độ 4bit
- Hỗ trợ nhiều kích thước LCD sử dụng chip HD44780
- Hiển thị được 8 ký tự đặc biệt
- Hiển thị con trỏ của LCD (cursor blinking)
- Tự dộng dịch dữ liệu trong ramShift content in ram left/right
- Tự động xuống dòng, khi có ký tự \n, \r hoặc \n\r
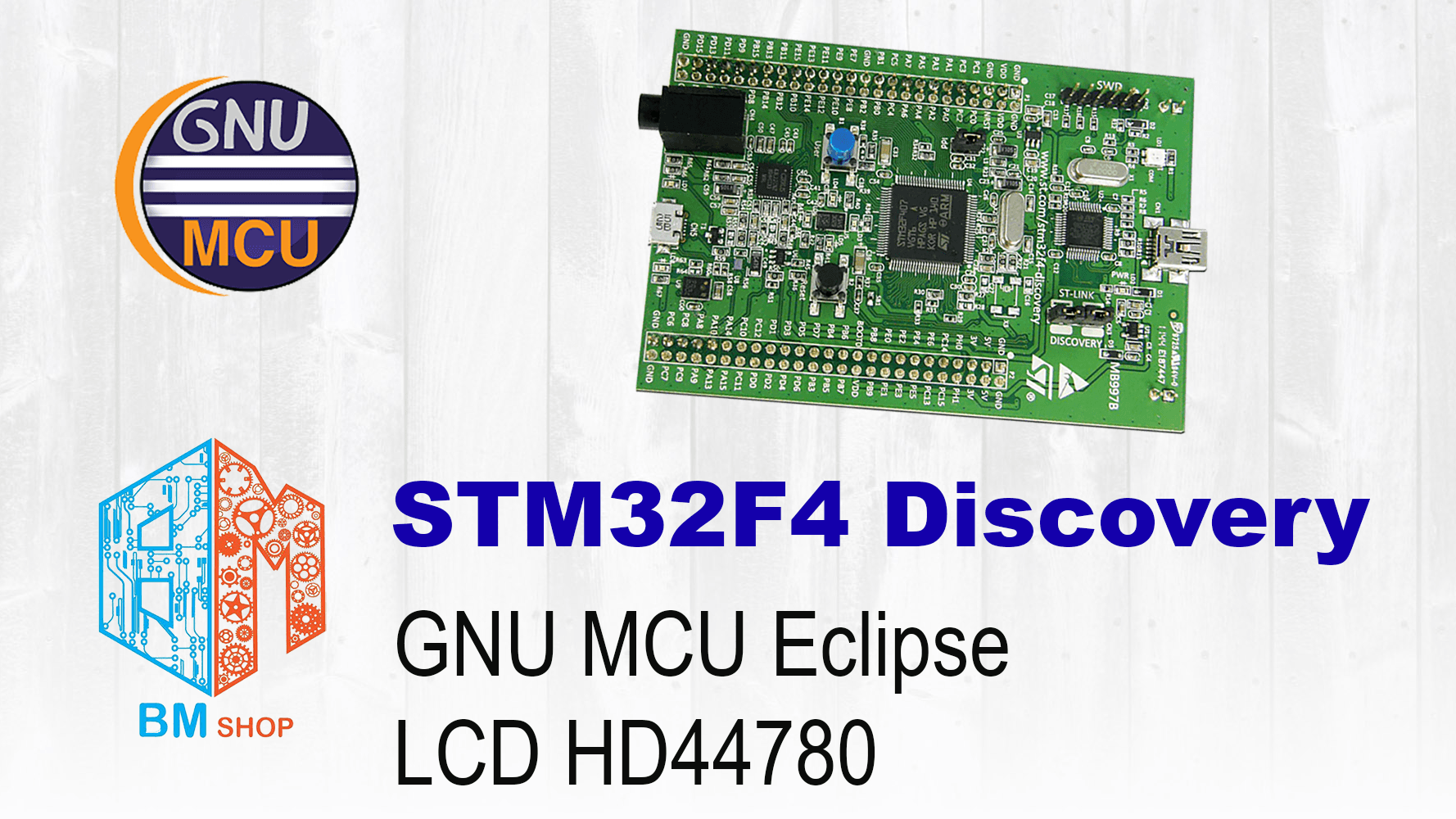
Software và Hardware sử dụng
- GNU MCU Eclipse
- Kit STM32F4 Discovery đã được cài Firmware hỗ trợ J-link debug
- LCD 16x2
- Dây cắm
- Biến trở
Sơ đồ đấu dây
- GND - GND
- VCC - +5V
- V0 - Biến trở
- RS - PB2
- RW - GND
- E - PB7
- D4 - PC12
- D5 - PC13
- D6 - PB12
- D7 - PB13
- A - +3V3
- K - GND
Kết quả
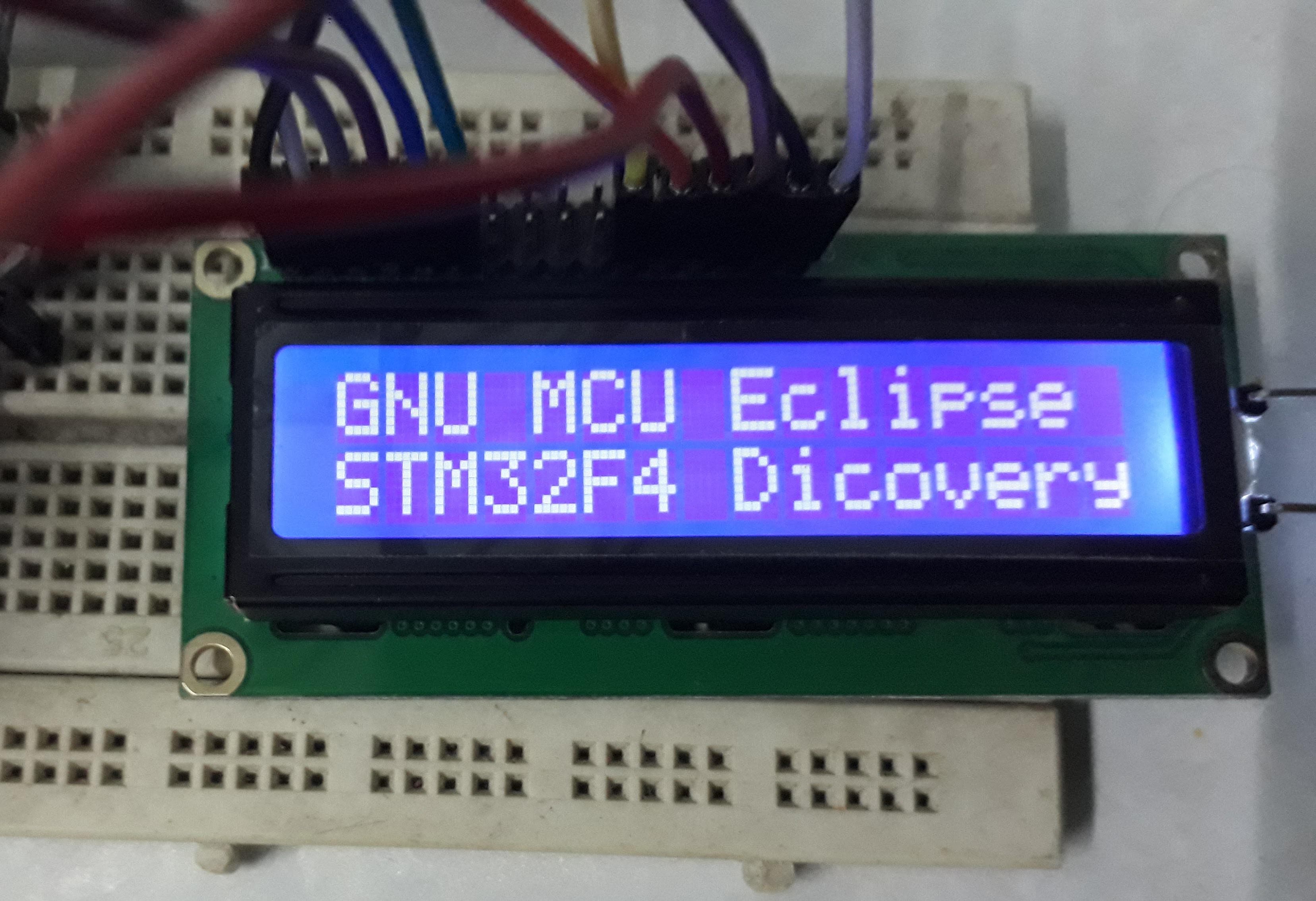
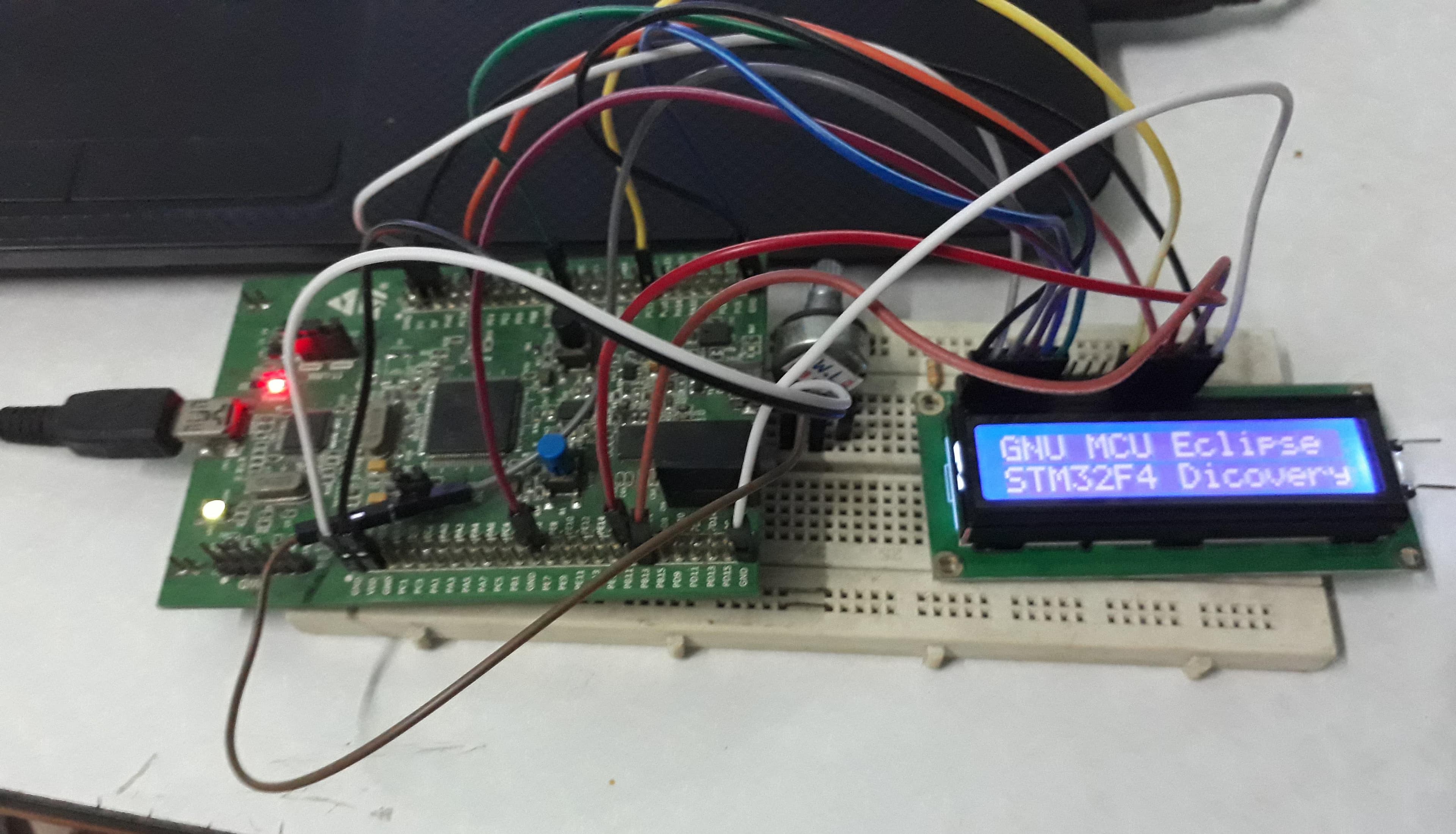
Code main.c
/**
* GNU MCU Eclipse project example for HD44780
*
* Before you start, select your target, on the right of the "Load" button
*
* @author nvtienanh
* @email nvtienanh@gmail.com
* @website https://bmshop.vn
* @ide GNU MCU Eclipse
* @stdperiph STM32F4xx/STM32F7xx HAL drivers required
*/
/* Include core modules */
#include <stdio.h>
#include <stdlib.h>
#include "diag/Trace.h"
#include "stm32fxxx_hal.h"
#include "defines.h"
#include "stm32_disco.h"
#include "stm32_delay.h"
#include "stm32_hd44780.h"
#include "stm32_general.h"
// ----- main() ---------------------------------------------------------------
// Sample pragmas to cope with warnings. Please note the related line at
// the end of this function, used to pop the compiler diagnostics status.
#pragma GCC diagnostic push
#pragma GCC diagnostic ignored "-Wunused-parameter"
#pragma GCC diagnostic ignored "-Wmissing-declarations"
#pragma GCC diagnostic ignored "-Wreturn-type"
/* String variable for LCD */
uint8_t customChar[] = {
0x1F, // xxx 11111
0x11, // xxx 10001
0x11, // xxx 10001
0x11, // xxx 10001
0x11, // xxx 10001
0x11, // xxx 10001
0x11, // xxx 10001
0x1F // xxx 11111
};
int main(int argc, char* argv[])
{
/* Init system clock for maximum system speed */
RCC_InitSystem();
/* Init HAL layer */
HAL_Init();
/* Init leds */
DISCO_LedInit();
/* Init button */
DISCO_ButtonInit();
/* Init LCD */
//Initialize LCD 16 cols x 2 rows
HD44780_Init(16, 2);
//Save custom character on location 0 in LCD
HD44780_CreateChar(0, &customChar[0]);
//Put string to LCD
HD44780_Puts(0, 0, "GNU MCU Eclipse");
HD44780_Puts(0, 1, "STM32F4 Dicovery");
//Wait a little
Delayms(5000);
//Clear LCD
HD44780_Clear();
//Show cursor
HD44780_CursorOn();
//Write new text
HD44780_Puts(0, 0, "CLEARED!");
//Wait a little
Delayms(1000);
//Enable cursor blinking
HD44780_BlinkOn();
//Show custom character at x = 1, y = 2 from RAM location 0
HD44780_PutCustom(1, 1, 0);
while (1)
{
/*
#ifdef TRACE
trace_printf("Device error!\r\n");
#else
printf("Device error!\r\n");
#endif
*/
}
}